Triangles and Z-Buffer
Last updated on 8 months ago
投影矩阵
透视投影矩阵推导
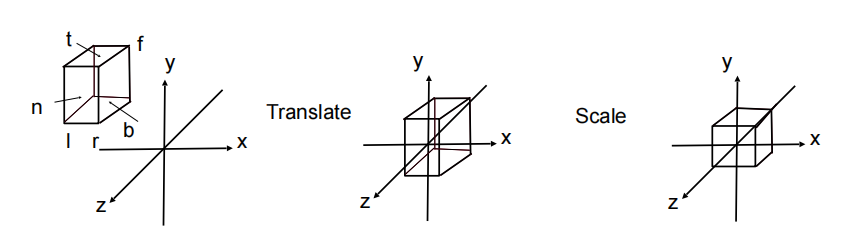
对于正交投影(Orthographic Projection)需要把长方体
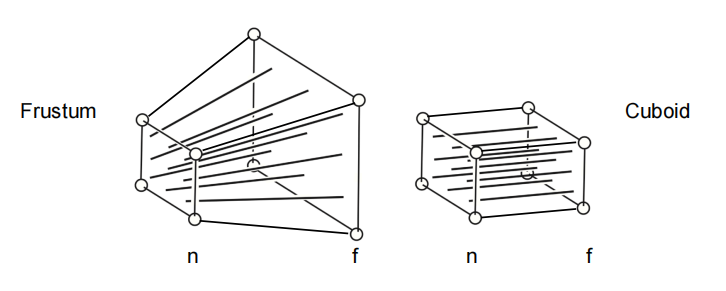
对于透视投影(Perspective Projection),思路是把视锥体“挤压”成长方体,再对长方体进行归一化。于是视锥体内的所有点“挤压”到近平面上(可以想象视角在远平面外侧,方向垂直于远平面),可以根据相似三角形原理计算出“挤压”后的
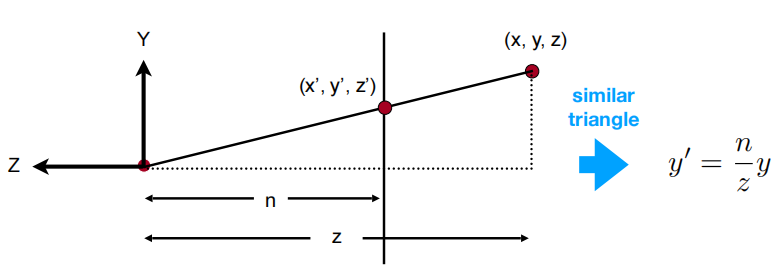
那么“挤压”后的点在齐次坐标系(Homogeneous Coordinates)下可以表示为:
所以从视锥体到长方体的矩阵变换
即
第三行的未知值可以根据两个设定计算出来:
近平面上的任意一点经过“挤压”后的
坐标不发生变化,近平面上的点为 ,挤压后还是 ,也可以写成 ,所以第三行一定满足以下等式:
可以得出:远平面上的任意一点经过“挤压”后的
坐标也不会发生变化,同上可得:
联立可得:
所以透视投影矩阵可以表示为:
对于函数 get_projection_matrix
中的参数可由下图计算出:
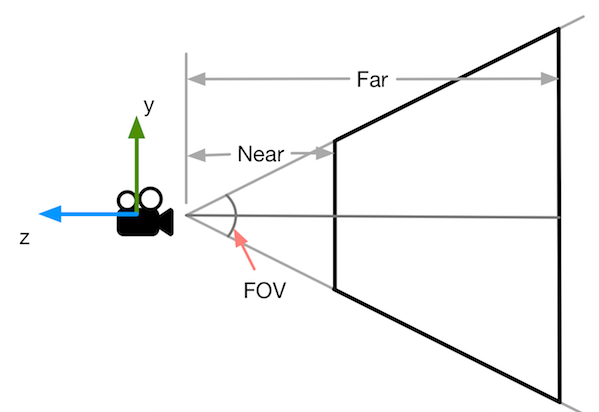
长方体的中心位于原点,则
旋转与投影代码
1 |
|
三维空间中判断一个点是否在三角形内
- 求出向量
, , - 计算
, , - 如果叉乘后三个向量方向同向则说明
点在三角形内部,否则在外部
1 |
|
光栅化
计算重心坐标
考虑三角形三个顶点
解方程组可得
1 |
|
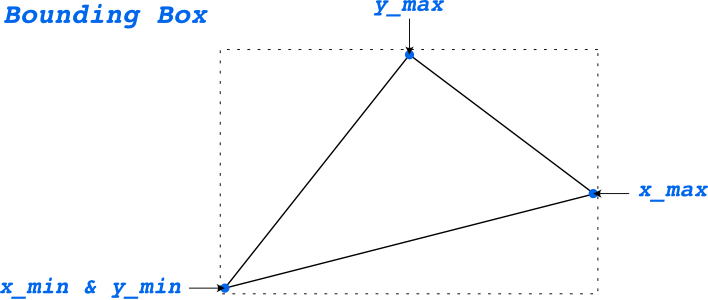
光栅化一个三角形需要扫描三角形所在的包围盒,判断点是否在三角形内部然后通过中心坐标插值
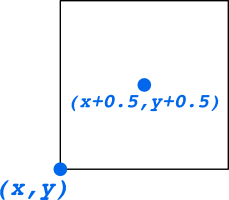
所以判断是否在三角形内部需要加
1 |
|
已知重心坐标
其中
重心坐标是在 2D 空间里做的,不能用作插值 3D 空间的坐标,需要经过插值矫正才可以
1 |
|